mirror of
https://github.com/paperless-ngx/paperless-ngx.git
synced 2025-04-02 13:45:10 -05:00
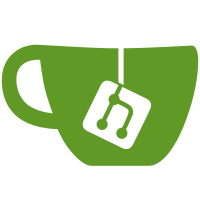
* Implement share links Basic implementation of share links Make certain share link fields not editable, automatically grant permissions on migrate Updated styling, error messages from expired / deleted links frontend code linting, reversable sharelink migration testing coverage Update translation strings No links message * Consolidate file response methods * improvements to share links on mobile devices * Refactor share links file_version * Add docs for share links * Apply suggestions from code review * When filtering share links, use the timezone aware now() * Removes extra call to setup directories for usage in testing * FIx copied badge display on some browsers * Move copy to ngx-clipboard library --------- Co-authored-by: Trenton H <797416+stumpylog@users.noreply.github.com>
43 lines
1.4 KiB
TypeScript
43 lines
1.4 KiB
TypeScript
import { HttpTestingController } from '@angular/common/http/testing'
|
|
import { TestBed } from '@angular/core/testing'
|
|
import { Subscription } from 'rxjs'
|
|
import { environment } from 'src/environments/environment'
|
|
import { commonAbstractPaperlessServiceTests } from './abstract-paperless-service.spec'
|
|
import { ShareLinkService } from './share-link.service'
|
|
|
|
let httpTestingController: HttpTestingController
|
|
let service: ShareLinkService
|
|
let subscription: Subscription
|
|
const endpoint = 'share_links'
|
|
|
|
// run common tests
|
|
commonAbstractPaperlessServiceTests(endpoint, ShareLinkService)
|
|
|
|
describe(`Additional service tests for ShareLinkService`, () => {
|
|
beforeEach(() => {
|
|
// Dont need to setup again
|
|
|
|
httpTestingController = TestBed.inject(HttpTestingController)
|
|
service = TestBed.inject(ShareLinkService)
|
|
})
|
|
|
|
afterEach(() => {
|
|
subscription?.unsubscribe()
|
|
httpTestingController.verify()
|
|
})
|
|
|
|
it('should support creating link for document', () => {
|
|
subscription = service.createLinkForDocument(0).subscribe()
|
|
httpTestingController
|
|
.expectOne(`${environment.apiBaseUrl}${endpoint}/`)
|
|
.flush({})
|
|
})
|
|
|
|
it('should support get links for a document', () => {
|
|
subscription = service.getLinksForDocument(0).subscribe()
|
|
httpTestingController
|
|
.expectOne(`${environment.apiBaseUrl}documents/0/${endpoint}/`)
|
|
.flush({})
|
|
})
|
|
})
|