mirror of
https://github.com/paperless-ngx/paperless-ngx.git
synced 2025-04-02 13:45:10 -05:00
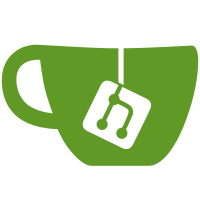
This commit adds a `Dockerfile` to the root of the project, accompanied by a `docker-compose.yml.example` for simplified deployment. The `Dockerfile` is agnostic to whether it will be the webserver, the consumer, or if it is run for a one-off command (i.e. creation of a superuser, migration of the database, document export, ...). The containers entrypoint is the `scripts/docker-entrypoint.sh` script. This script verifies that the required permissions are set, remaps the default users and/or groups id if required and installs additional languages if the user wishes to. After initialization, it analyzes the command the user supplied: - If the command starts with a slash, it is expected that the user wants to execute a binary file and the command will be executed without further intervention. (Using `exec` to effectively replace the started shell-script and not have any reaping-issues.) - If the command does not start with a slash, the command will be passed directly to the `manage.py` script without further modification. (Again using `exec`.) The default command is set to `--help`. If the user wants to execute a command that is not meant for `manage.py` but doesn't start with a slash, the Docker `--entrypoint` parameter can be used to circumvent the mechanics of `docker-entrypoint.sh`. Further information can be found in `docs/setup.rst` and in `docs/migrating.rst`. For additional convenience, a `Dockerfile` has been added to the `docs/` directory which allows for easy building and serving of the documentation. This is documented in `docs/requirements.rst`.
75 lines
1.8 KiB
Bash
75 lines
1.8 KiB
Bash
#!/bin/bash
|
|
set -e
|
|
|
|
# Source: https://github.com/sameersbn/docker-gitlab/
|
|
map_uidgid() {
|
|
USERMAP_ORIG_UID=$(id -u paperless)
|
|
USERMAP_ORIG_UID=$(id -g paperless)
|
|
USERMAP_GID=${USERMAP_GID:-${USERMAP_UID:-$USERMAP_ORIG_GID}}
|
|
USERMAP_UID=${USERMAP_UID:-$USERMAP_ORIG_UID}
|
|
if [[ ${USERMAP_UID} != ${USERMAP_ORIG_UID} || ${USERMAP_GID} != ${USERMAP_ORIG_GID} ]]; then
|
|
echo "Mapping UID and GID for paperless:paperless to $USERMAP_UID:$USERMAP_GID"
|
|
groupmod -g ${USERMAP_GID} paperless
|
|
sed -i -e "s|:${USERMAP_ORIG_UID}:${USERMAP_GID}:|:${USERMAP_UID}:${USERMAP_GID}:|" /etc/passwd
|
|
fi
|
|
}
|
|
|
|
set_permissions() {
|
|
# Set permissions for consumption directory
|
|
chgrp paperless "$PAPERLESS_CONSUME"
|
|
chmod g+x "$PAPERLESS_CONSUME"
|
|
|
|
# Set permissions for application directory
|
|
chown -Rh paperless:paperless /usr/src/paperless
|
|
}
|
|
|
|
initialize() {
|
|
map_uidgid
|
|
set_permissions
|
|
}
|
|
|
|
install_languages() {
|
|
local langs="$1"
|
|
read -ra langs <<<"$langs"
|
|
|
|
# Check that it is not empty
|
|
if [ ${#langs[@]} -eq 0 ]; then
|
|
return
|
|
fi
|
|
|
|
# Update apt-lists
|
|
apt-get update
|
|
|
|
# Loop over languages to be installed
|
|
for lang in "${langs[@]}"; do
|
|
pkg="tesseract-ocr-$lang"
|
|
if dpkg -s "$pkg" 2>&1 > /dev/null; then
|
|
continue
|
|
fi
|
|
|
|
if ! apt-cache show "$pkg" 2>&1 > /dev/null; then
|
|
continue
|
|
fi
|
|
|
|
apt-get install "$pkg"
|
|
done
|
|
|
|
# Remove apt lists
|
|
rm -rf /var/lib/apt/lists/*
|
|
}
|
|
|
|
|
|
if [[ "$1" != "/"* ]]; then
|
|
initialize
|
|
|
|
# Install additional languages if specified
|
|
if [ ! -z "$PAPERLESS_OCR_LANGUAGES" ]; then
|
|
install_languages "$PAPERLESS_OCR_LANGUAGES"
|
|
fi
|
|
|
|
exec sudo -HEu paperless "/usr/src/paperless/src/manage.py" "$@"
|
|
fi
|
|
|
|
exec "$@"
|
|
|