mirror of
https://github.com/paperless-ngx/paperless-ngx.git
synced 2025-04-02 13:45:10 -05:00
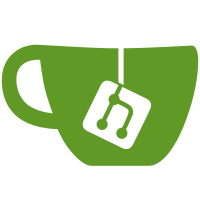
* Support all URI schemes * Reworks custom field value validation to check and return a 400 error code in more cases and support more URL looking items, not just some basic schemes * Fixes a spelling error in the message --------- Co-authored-by: Trenton H <797416+stumpylog@users.noreply.github.com>
30 lines
971 B
Python
30 lines
971 B
Python
from urllib.parse import urlparse
|
|
|
|
from django.core.exceptions import ValidationError
|
|
from django.utils.translation import gettext_lazy as _
|
|
|
|
|
|
def uri_validator(value) -> None:
|
|
"""
|
|
Raises a ValidationError if the given value does not parse as an
|
|
URI looking thing, which we're defining as a scheme and either network
|
|
location or path value
|
|
"""
|
|
try:
|
|
parts = urlparse(value)
|
|
if not parts.scheme:
|
|
raise ValidationError(
|
|
_(f"Unable to parse URI {value}, missing scheme"),
|
|
params={"value": value},
|
|
)
|
|
elif not parts.netloc and not parts.path:
|
|
raise ValidationError(
|
|
_(f"Unable to parse URI {value}, missing net location or path"),
|
|
params={"value": value},
|
|
)
|
|
except Exception as e:
|
|
raise ValidationError(
|
|
_(f"Unable to parse URI {value}"),
|
|
params={"value": value},
|
|
) from e
|